Description
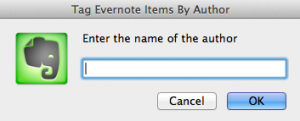
Do you use Evernote's email feature to have receipts or email notifications sent directly to your Evernote notebook? (Brett Kelly's eBook, "Evernote Essentials" provides a solid guide to how this works -- along with some other great tips -- in the chapter called "Evernote, Email and You" )
Inspired by this question on Quora, I whipped up this script which quickly tags or untags all items created by a selected "author" (for example, a note with a Quora email return address).)
INSTRUCTIONS
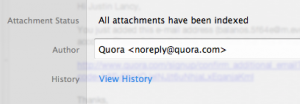
This script looks for the Author Name in the Clipboard, so you can speed things up by first selecting a note you know was created by the "Author" you're looking for. Look inside the Note's information (the lowercase "i" in the note window) and then select and copy the Author Name to the Clipboard before running the script. (Of course, you can type it in as well). If the script finds notes matching that Author name, you'll then be able to type in the tags you'd like to add or remove from those items!
The Code
Veritrope.com
Evernote - Author Tagger
Version 1.0
January 28, 2012
// SCRIPT INFORMATION AND UPDATE PAGE
http://veritrope.com/code/evernote-tag-author
// REQUIREMENTS
More details on the script information page.
//CHANGELOG
1.0 INITIAL RELEASE
// RECOMMENDED INSTALLATION INSTRUCTIONS:
This script looks for the Author Name in the Clipboard, so copy the Author Name to it before
running the script. (You can type it in as well). If it finds notes matching that name,
you can type in the tags you'd like to add or remove from those items!
*)
(*
======================================
// OTHER PROPERTIES (USE CAUTION WHEN CHANGING)
======================================
*)
property DialogTitle : "Tag Evernote Items By Author"
(*
======================================
// MAIN PROGRAM
======================================
*)
set defaultTag to the clipboard
--ENTER AUTHOR NAME (DEFAULT: CLIPBOARD)
set author_Result to (display dialog "Enter the name of the author" with title DialogTitle default answer defaultTag with icon path to resource "Evernote.icns" in bundle (path to application "Evernote"))
set author_Name to (text returned of author_Result)
set author_Search to ("author:"" & author_Name & """) as string
set defaultTag to ""
--GET LIST OF ITEMS MATCHING AUTHOR NAME
tell application "Evernote"
set noteList to find notes (author_Search)
end tell
--IF ITEMS FOUND, THEN ENTER TAGS
if (count of noteList) is greater than 0 then
display dialog "Enter Tags You'd Like To Assign or Remove Separated By Commas" with title DialogTitle default answer defaultTag buttons {"Assign", "Remove", "Cancel"} default button "Assign" cancel button "Cancel" with icon path to resource "Evernote.icns" in bundle (path to application "Evernote")
set dialogresult to the result
set userInput to text returned of dialogresult
set ButtonSel to button returned of dialogresult
set theDelims to {","}
--ASSEMBLE TAGS FROM INPUT
set EVTag to my Tag_List(userInput, theDelims)
--FINAL CHECK AND FORMATTING OF TAGS
set final_Tag_List to my Tag_Check(EVTag)
--ADD OR REMOVE THE TAGS
if ButtonSel is "Assign" then
tell application "Evernote"
assign final_Tag_List to noteList
end tell
else if ButtonSel is "Remove" then
tell application "Evernote"
unassign final_Tag_List from noteList
end tell
end if
--ELSE IF ITEMS NOT FOUND...
else
display dialog "No Items Found for Author!" with title DialogTitle with icon path to resource "Evernote.icns" in bundle (path to application "Evernote")
end if
(*
======================================
/// TAGGING SUBROUTINES
======================================
*)
--CREATES TAGS LIST
on Tag_List(userInput, theDelims)
set oldDelims to AppleScript's text item delimiters
set theList to {userInput}
repeat with aDelim in theDelims
set AppleScript's text item delimiters to aDelim
set newList to {}
repeat with anItem in theList
set newList to newList & text items of anItem
end repeat
set theList to newList
end repeat
return theList as list
set AppleScript's text item delimiters to oldDelims
end Tag_List
--CREATES TAGS IF THEY DON'T EXIST
on Tag_Check(theTags)
tell application "Evernote"
set finalTags to {}
repeat with theTag in theTags
if (not (tag named theTag exists)) then
set makeTag to make tag with properties {name:theTag}
set end of finalTags to makeTag
else
set end of finalTags to tag theTag
end if
end repeat
end tell
return finalTags
end Tag_Check